Top 5 Common Issues When Using GraphQL with React, Apollo Client, and Auth0 (And How to Solve Them)
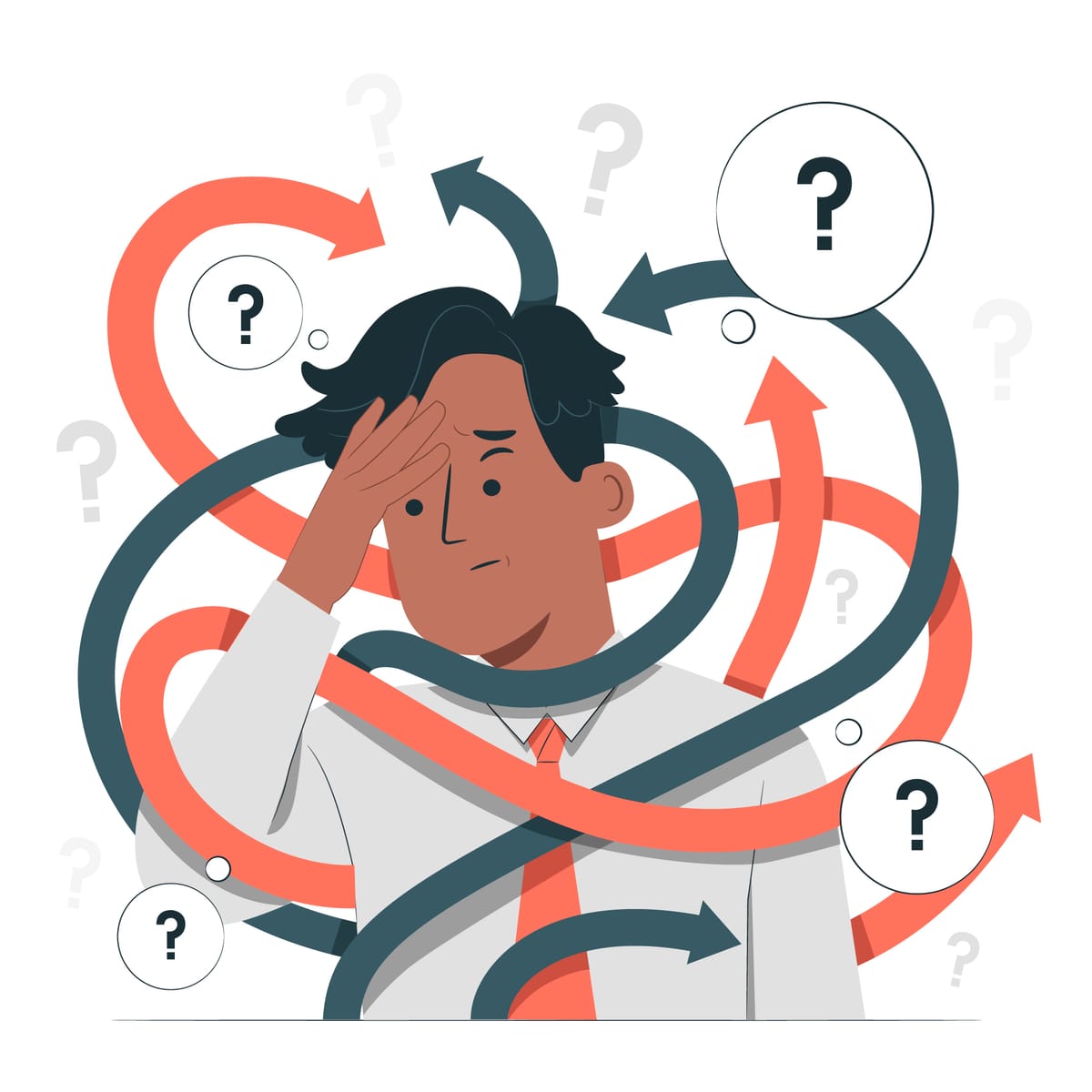
GraphQL has redefined how developers build and consume APIs, offering unmatched flexibility and precision compared to REST. Paired with React and Apollo Client, it powers dynamic, data-driven applications with ease. Throw Auth0 into the mix for authentication and authorization, and you’ve got a modern stack that’s secure and scalable. Yet, this powerful combination comes with its own set of challenges. From query complexity to debugging woes, developers often hit roadblocks that can slow progress or frustrate users.
In this blog, we’ll explore the top five common issues when using GraphQL with a React app powered by Apollo Client and secured with Auth0. For each, I’ll share practical solutions, code examples, and a few insights drawn from real-world experience to help you overcome these hurdles and build better applications. Let’s jump in!
1. Managing Complex Query States
The Issue
Apollo Client’s useQuery hook simplifies data fetching, but in complex applications, juggling loading, error, and data states across components can lead to messy, repetitive code. Unhandled edge cases — like network timeouts or partial responses — can also slip through the cracks.
The Solution
Centralize state management with custom hooks or tap into Apollo’s features like fetchPolicy and error handling to keep your code DRY (Don’t Repeat Yourself) and robust.
Code Example
import { useQuery, gql } from '@apollo/client';
const GET_USER_PROFILE = gql`
query GetUserProfile($userId: ID!) {
user(id: $userId) {
id
name
email
}
}
`;
const useUserProfile = (userId) => {
const { data, loading, error, refetch } = useQuery(GET_USER_PROFILE, {
variables: { userId },
fetchPolicy: 'cache-and-network',
onError: (err) => {
console.error('Query failed:', err.message);
},
});
return {
user: data?.user,
isLoading: loading,
error: error?.message,
retry: refetch,
};
};
// Usage in a component
const UserProfile = ({ userId }) => {
const { user, isLoading, error, retry } = useUserProfile(userId);
if (isLoading) return <p>Loading...</p>;
if (error) return <p>Error: {error} <button onClick={retry}>Retry</button></p>;
return <div>Hello, {user.name} ({user.email})</div>;
};
Explanation
This custom hook wraps query logic for reusability. The fetchPolicy balances cache usage with fresh data, while onError ensures errors are caught early.
Insight
In practice, over-relying on loading states can frustrate users if your API is slow. Consider adding skeleton screens or optimistic UI updates (via Apollo’s update function) to improve perceived performance. Also, test with flaky networks — tools like Chrome’s DevTools throttling can reveal hidden state-handling bugs.
2. Overfetching or Underfetching Data
The Issue
GraphQL’s strength is fetching exactly what you need, but misaligned queries can cause overfetching (grabbing excess data) or underfetching (missing key fields). This often creeps in as components evolve faster than their queries.
The Solution
Use GraphQL fragments to enforce consistency and periodically audit queries with Apollo’s dev tools to match your UI’s needs.
Code Example
import { gql } from '@apollo/client';
const USER_FRAGMENT = gql`
fragment UserDetails on User {
id
name
email
profilePicture
}
`;
const GET_USER_WITH_POSTS = gql`
query GetUserWithPosts($userId: ID!) {
user(id: $userId) {
...UserDetails
posts {
id
title
}
}
}
${USER_FRAGMENT}
`;
Explanation
Fragments ensure reusable, consistent field sets. A leaner query can reuse UserDetails without fetching posts if unneeded.
Insight
Overfetching often sneaks in when teams rush features without schema reviews. I’ve seen apps fetch entire user profiles for a simple avatar display — wasting bandwidth and slowing renders. Set up a process to pair query updates with PR reviews, and use tools like GraphQL Inspector to catch schema drift early.
3. Handling Authorization Errors
The Issue
GraphQL APIs can return authorization errors (e.g., 403) when users lack permission, even with valid authentication. Apollo Client doesn’t natively separate these from other errors, leaving you to handle them manually.
The Solution
Add a custom error link to intercept authorization issues and provide user-friendly feedback or redirects.
Code Example
import { onError } from '@apollo/client/link/error';
import { ApolloClient, InMemoryCache, HttpLink } from '@apollo/client';
const errorLink = onError(({ graphQLErrors, networkError }) => {
if (graphQLErrors) {
graphQLErrors.forEach(({ message, extensions }) => {
if (extensions?.code === 'FORBIDDEN') {
alert('You don't have permission to perform this action.');
}
});
}
if (networkError) {
console.log(`Network error: ${networkError}`);
}
});
const httpLink = new HttpLink({
uri: '<https://your-graphql-api-endpoint.com/graphql>',
});
const client = new ApolloClient({
link: errorLink.concat(httpLink),
cache: new InMemoryCache(),
});
Explanation
The errorLink catches FORBIDDEN errors (custom to your API) and alerts users, keeping the experience smooth.
Insight
Authorization errors often spike when roles or permissions change on the backend. I’ve found it helpful to log these errors to a service like Sentry with user context — this reveals patterns (e.g., outdated UI assumptions) and speeds up fixes. Also, consider a global error boundary in React to catch unhandled cases gracefully.
4. Performance Issues with Large Queries
The Issue
Large or nested GraphQL queries can tank performance in growing React apps, causing slow renders or excessive data fetching. Apollo’s cache mitigates this, but unoptimized queries still drag things down.
The Solution
Implement pagination, lazy queries, and batching. Apollo’s useLazyQuery and @connection directive optimize data loading.
Code Example
import { useLazyQuery, gql } from '@apollo/client';
const GET_POSTS = gql`
query GetPosts($cursor: String) {
posts(first: 10, after: $cursor) @connection(key: "posts") {
edges {
node {
id
title
}
}
pageInfo {
endCursor
hasNextPage
}
}
}
`;
const PostList = () => {
const [loadPosts, { data, loading, fetchMore }] = useLazyQuery(GET_POSTS);
const loadMore = () => {
fetchMore({
variables: { cursor: data.posts.pageInfo.endCursor },
});
};
return (
<div>
<button onClick={() => loadPosts()}>Load Posts</button>
{loading && <p>Loading...</p>}
{data?.posts.edges.map(({ node }) => (
<p key={node.id}>{node.title}</p>
))}
{data?.posts.pageInfo.hasNextPage && <button onClick={loadMore}>More</button>}
</div>
);
};
Explanation
Lazy loading and pagination fetch data incrementally, while @connection ensures proper cache handling.
Insight
Performance issues often hide until production traffic hits. I once debugged a dashboard that loaded 500+ records at once — pagination cut load times from 8 seconds to under 2. Test with realistic data volumes early, and profile renders with React DevTools to catch re-render culprits tied to query results.
5. Difficulty Debugging GraphQL Queries and Mutations
The Issue
Debugging GraphQL can feel like finding a needle in a haystack — vague errors, single-endpoint ambiguity, and cache quirks make it tough to trace problems compared to REST’s explicit endpoints.
The Solution
Use Apollo Client DevTools, detailed logging, and isolated query testing (e.g., via GraphiQL) to pinpoint issues faster.
Code Example
import { useQuery, gql } from '@apollo/client';
const GET_USER_DATA = gql`
query GetUserData($id: ID!) {
user(id: $id) {
id
name
email
}
}
`;
const UserData = ({ userId }) => {
const { data, loading, error } = useQuery(GET_USER_DATA, {
variables: { id: userId },
onCompleted: (result) => {
console.log('Query completed:', result);
},
onError: (err) => {
console.error('Detailed error:', JSON.stringify(err, null, 2));
},
});
if (loading) return <p>Loading...</p>;
if (error) return <p>Error: Check console for details</p>;
return <div>{data.user.name} ({data.user.email})</div>;
};
Explanation
onCompleted and onError log detailed responses, while Apollo Client DevTools tracks cache and query execution.
Insight
GraphQL’s flexibility can mask typos or schema mismatches until runtime. I’ve wasted hours on a missing field that GraphiQL caught in seconds — always test queries outside your app first. Also, watch for cache-related bugs; a stale cache once showed outdated user roles in an app I built, fixed only after tweaking fetchPolicy.
GraphQL with React, Apollo Client, and Auth0 is a dream stack for modern apps, but it’s not without its quirks. Whether it’s wrangling query states, optimizing performance, or debugging mysteries, these five issues test every developer. With custom hooks, fragments, error links, pagination, and smart debugging, you can conquer them and ship a polished product.
These solutions, paired with the insights shared, draw from real-world lessons to save you time and headaches. Hopefully it solves some of your issues!