Real-Time Data Analytics with Kafka Streams: A Beginner's Guide
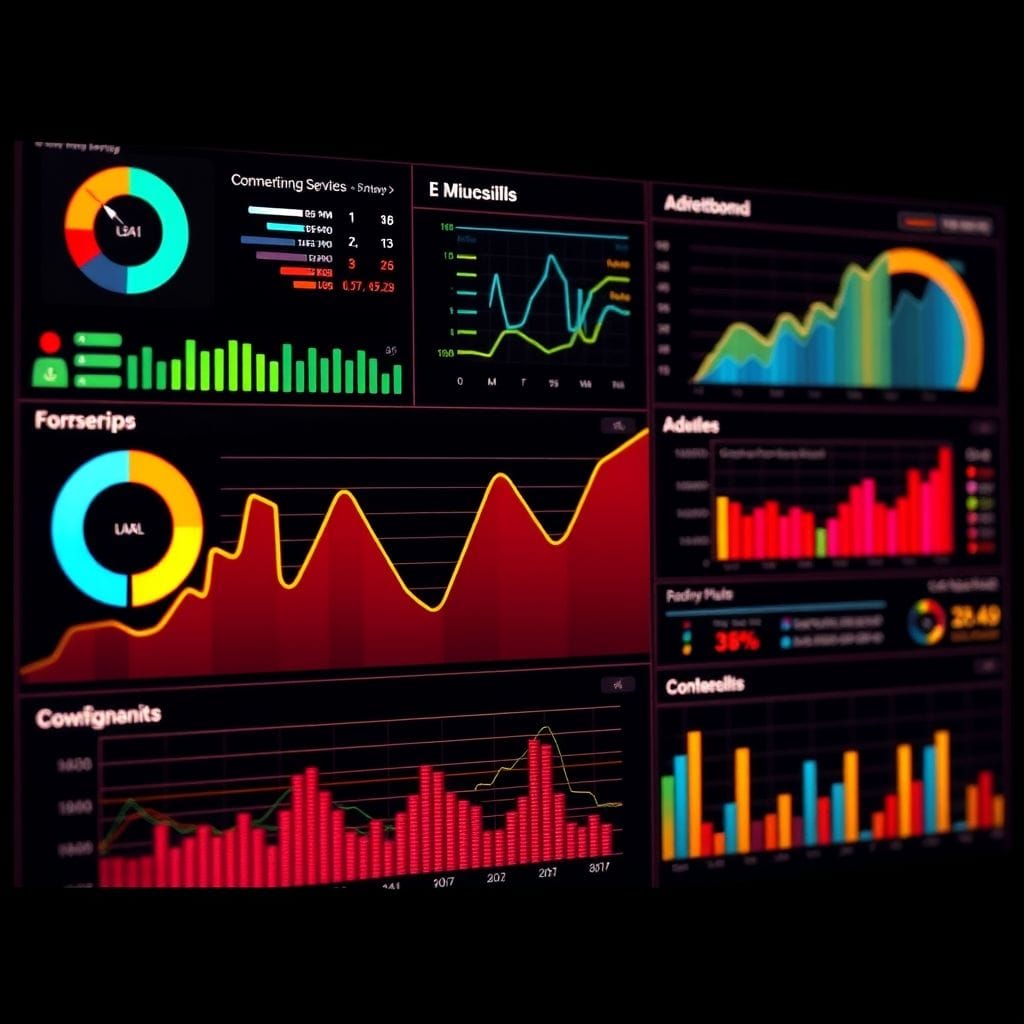
Introduction
In today's data-driven world, processing information in real-time has become crucial for businesses. At Posit Source Technologies Private Limited, we've helped numerous organizations implement real-time analytics using Kafka Streams. Let's explore how you can leverage this powerful technology.
Understanding Kafka Streams
Kafka Streams is a client library for building applications and microservices that process and analyze data stored in Kafka. Think of it as a Swiss Army knife for real-time data processing.
Basic Concepts
// Example of a simple Kafka Streams application
import org.apache.kafka.streams.StreamsBuilder;
import org.apache.kafka.streams.kstream.KStream;
public class SimpleStreamProcessor {
public static void main(String[] args) {
StreamsBuilder builder = new StreamsBuilder();
// Read from input topic
KStream<String, String> inputStream = builder.stream("input-topic");
// Process the stream
KStream<String, String> processedStream = inputStream
.filter((key, value) -> value != null)
.mapValues(value -> value.toUpperCase());
// Write to output topic
processedStream.to("output-topic");
}
}
Real-World Use Case: E-Commerce Analytics
Let's look at a practical example: real-time sales analytics for an e-commerce platform.
public class EcommerceAnalytics {
public static void main(String[] args) {
Properties config = new Properties();
config.put(StreamsConfig.APPLICATION_ID_CONFIG, "ecommerce-analytics");
config.put(StreamsConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
StreamsBuilder builder = new StreamsBuilder();
// Create stream from sales topic
KStream<String, Order> orderStream = builder.stream("sales",
Consumed.with(Serdes.String(), orderSerde));
// Calculate revenue per category
KTable<String, Double> revenueByCategory = orderStream
.groupBy((key, order) -> order.getCategory(),
Grouped.with(Serdes.String(), orderSerde))
.aggregate(
() -> 0.0,
(key, order, total) -> total + order.getAmount(),
Materialized.with(Serdes.String(), Serdes.Double())
);
// Create 5-minute sales window
TimeWindows window = TimeWindows.of(Duration.ofMinutes(5));
KTable<Windowed<String>, Double> salesWindow = orderStream
.groupByKey()
.windowedBy(window)
.aggregate(
() -> 0.0,
(key, order, total) -> total + order.getAmount()
);
}
}
Advanced Pattern: Fraud Detection
Here's how we implemented real-time fraud detection:
public class FraudDetection {
public static void main(String[] args) {
StreamsBuilder builder = new StreamsBuilder();
// Create transaction stream
KStream<String, Transaction> transactions = builder
.stream("transactions",
Consumed.with(Serdes.String(), transactionSerde));
// Create 5-minute sliding window
TimeWindows window = TimeWindows.of(Duration.ofMinutes(5))
.advanceBy(Duration.ofMinutes(1));
// Detect suspicious patterns
KTable<Windowed<String>, Long> suspiciousActivity = transactions
.groupByKey()
.windowedBy(window)
.count()
.filter((key, count) -> count > 10); // Threshold
// Alert on suspicious activity
suspiciousActivity.toStream()
.map((key, count) -> KeyValue.pair(
key.key(),
createAlert(key.key(), count)
))
.to("fraud-alerts");
}
}
Best Practices from Our Experience
1. State Management
// Example of proper state store usage
public class StateManagement {
public static void main(String[] args) {
StreamsBuilder builder = new StreamsBuilder();
// Create state store
StoreBuilder<KeyValueStore<String, Long>> storeBuilder =
Stores.keyValueStoreBuilder(
Stores.persistentKeyValueStore("counts-store"),
Serdes.String(),
Serdes.Long()
);
builder.addStateStore(storeBuilder);
// Use state store in processing
KStream<String, String> input = builder.stream("input-topic");
input.process(() -> new Processor<String, String>() {
private KeyValueStore<String, Long> store;
@Override
public void init(ProcessorContext context) {
store = context.getStateStore("counts-store");
}
@Override
public void process(String key, String value) {
Long count = store.get(key);
if (count == null) count = 0L;
store.put(key, count + 1);
}
}, "counts-store");
}
}
2. Error Handling
public class ResilientProcessor {
public static void main(String[] args) {
KStream<String, String> input = builder.stream("input-topic");
// Handle errors gracefully
input.mapValues(value -> {
try {
return processValue(value);
} catch (Exception e) {
log.error("Error processing value: " + value, e);
return createErrorRecord(value, e);
}
})
.branch(
(key, value) -> value.isError(),
(key, value) -> !value.isError()
);
}
}
3. Performance Optimization
public class OptimizedProcessor {
public static void main(String[] args) {
Properties config = new Properties();
// Optimize for performance
config.put(StreamsConfig.CACHE_MAX_BYTES_BUFFERING_CONFIG, 10 * 1024 * 1024L);
config.put(StreamsConfig.COMMIT_INTERVAL_MS_CONFIG, 1000);
config.put(StreamsConfig.NUM_STREAM_THREADS_CONFIG, 3);
StreamsBuilder builder = new StreamsBuilder();
// ... stream processing logic
}
}
Monitoring and Maintenance
We've developed a comprehensive monitoring approach:
public class MonitoredProcessor {
public static void main(String[] args) {
// Add custom metrics
Metrics.addSensor(
"process-latency",
Sensor.RecordingLevel.INFO,
StreamsMetrics.LATENCY_METRIC
);
// Monitor state store
KafkaStreams streams = new KafkaStreams(builder.build(), config);
streams.setStateListener((newState, oldState) -> {
if (newState == KafkaStreams.State.ERROR) {
// Alert operations team
notifyOperations("Stream entered ERROR state");
}
});
}
}
Scaling Considerations
Here's how we handle scaling:
Partition Strategy:
public class CustomPartitioner implements StreamPartitioner<String, String> {
@Override
public Integer partition(String topic, String key, String value,
int numPartitions) {
// Custom partition logic
return Math.abs(key.hashCode()) % numPartitions;
}
}
Horizontal Scaling
config.put(StreamsConfig.NUM_STANDBY_REPLICAS_CONFIG, 1);
config.put(StreamsConfig.REPLICATION_FACTOR_CONFIG, 3);
Getting Started Guide
Create your first processor:
public class QuickStart {
public static void main(String[] args) {
StreamsBuilder builder = new StreamsBuilder();
KStream<String, String> source = builder.stream("quickstart-topic");
source.mapValues(value -> value.toUpperCase())
.to("output-topic");
}
}
Set up your development environment:
<dependency>
<groupId>org.apache.kafka</groupId>
<artifactId>kafka-streams</artifactId>
<version>3.4.0</version>
</dependency>
Contact Us
Ready to implement real-time analytics in your organization? Our team can help:
- Design scalable stream processing architectures
- Implement custom processors for your use case
- Optimize performance and reliability
- Provide ongoing support and maintenance
Let's discuss how we can help you leverage Kafka Streams for your real-time data needs.
Posit Source Technologies Private Limited specializes in real-time data processing solutions with extensive experience in Kafka Streams. Our team has successfully implemented stream processing solutions across various industries, helping organizations make better decisions with real-time data.