Exploring Micro-services in Full Stack Development with React.js
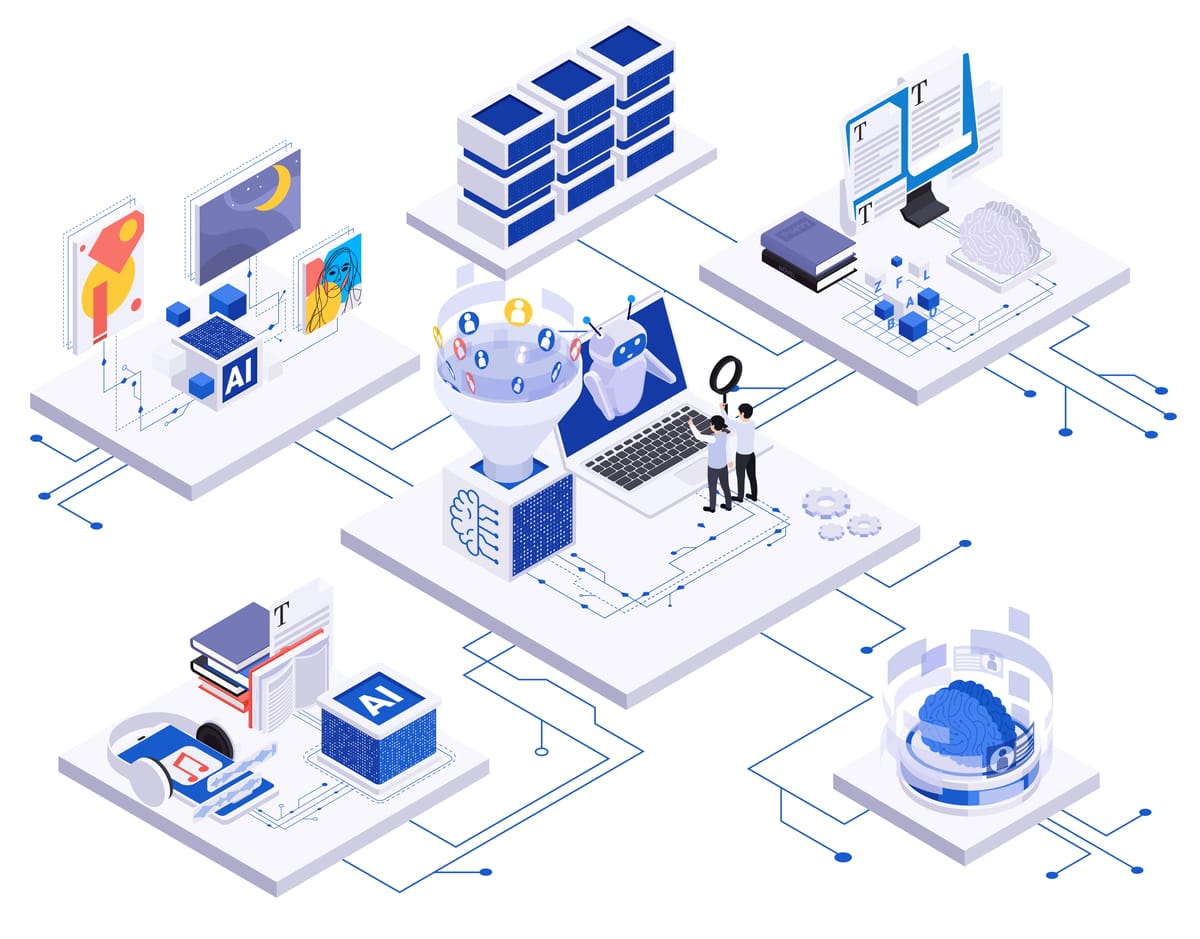
1. Introduction
In today’s fast-paced software development world, scalability, maintainability, and flexibility are key. Traditional monolithic architectures, where all functionalities exist within a single codebase, often struggle as applications grow. This is where microservices architecture comes in—breaking applications into smaller, independent services that communicate via APIs.
For full-stack developers, microservices revolutionize application development, enabling greater scalability and flexibility. While discussions often center on back-end technologies, the front-end is just as vital.
With its component-based structure and efficient data handling, React.js fits seamlessly into a microservices ecosystem. This blog explores how React interacts with microservices, offering practical insights and code examples to help you build scalable, full-stack applications.
2. Understanding Microservices Architecture
At its core, microservices architecture involves breaking down an application into loosely coupled, independently deployable services. Each service focuses on a specific business capability and can be developed, deployed, and scaled independently.
Key Principles and advantages of Microservices:
- Scalability: Individual services can be scaled based on demand.
- Fault Isolation: A failure in one service doesn’t bring down the entire application.
- Decentralized Data Management: Each service manages its own database, promoting autonomy.
- Independent Deployment: Services can be updated without redeploying the entire application.
- Polyglot Programming: Different microservices can be written in different programming languages.
- Improved Team Autonomy: Smaller, focused teams can own and manage individual microservices, fostering agility and ownership.
Monolithic vs. Microservices Architecture:
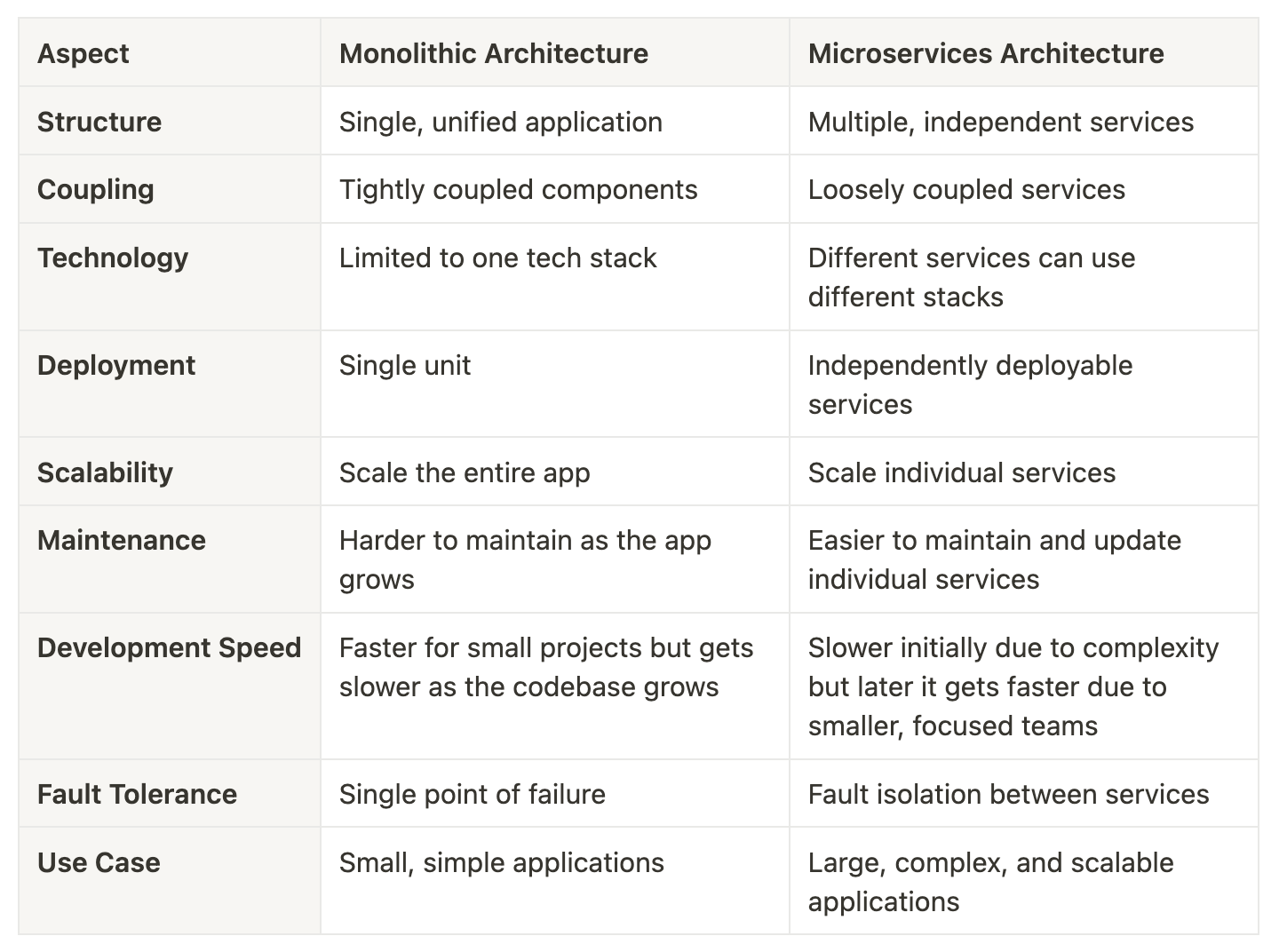
While microservices offer clear advantages, they also introduce complexities in service communication, data management, and front-end integration — areas where React shines.
3. How React.js Fits into Microservices Architecture
In a microservices environment, the front-end serves as the primary interface for users to engage with multiple services. React.js, known for its declarative and component-driven design, is an ideal choice for this purpose. Its modular structure aligns seamlessly with the decentralized nature of microservices, enabling efficient development and integration.
Why React Fits Well in Microservices:
- Component-Based Architecture: React’s modular approach mirrors the microservices philosophy, allowing developers to build reusable components that align with individual services.
- Efficient Data Handling: React’s virtual DOM and efficient state management make it ideal for handling dynamic data from multiple services.
- Scalability: As applications grow, React’s ecosystem (like Next.js for SSR, Redux for state management) supports scalability and maintainability.
Let’s take an example of a typical e-commerce platform built on microservices:
- User Service handles authentication.
- Product Service manages product listings.
- Order Service deals with transactions.
React acts as the orchestrator, pulling data from these services and presenting a seamless user experience.
4. Example of Microservices Architecture with React.js
In this example, we’ll create two microservices: Order Service and Inventory Service, and then build a React front-end to interact with these services. This example also includes a Gateway Service to aggregate data from the microservices.
Step 1: Creating Microservices with Node.js (Express.js)
- Order Service (order-service/index.js):
This service manages orders.
const express = require('express');
const app = express();
const PORT = 5001;
const orders = [
{ id: 1, productId: 101, quantity: 2 },
{ id: 2, productId: 102, quantity: 1 },
];
app.get('/orders', (req, res) => {
res.json(orders);
});
app.listen(PORT, () => {
console.log(`Order Service running on <http://localhost>:${PORT}`);
});
- Inventory Service (inventory-service/index.js)
This service manages product inventory.
const express = require('express');
const app = express();
const PORT = 5002;
const inventory = [
{ id: 101, name: 'Laptop', stock: 10 },
{ id: 102, name: 'Phone', stock: 20 },
];
app.get('/inventory', (req, res) => {
res.json(inventory);
});
app.listen(PORT, () => {
console.log(`Inventory Service running on <http://localhost>:${PORT}`);
});
- Gateway Service (gateway-service/index.js)
This service aggregates data from the Order and Inventory services.
const express = require('express');
const axios = require('axios');
const app = express();
const PORT = 5003;
app.get('/summary', async (req, res) => {
try {
const [ordersRes, inventoryRes] = await Promise.all([
axios.get('<http://localhost:5001/orders>'),
axios.get('<http://localhost:5002/inventory>'),
]);
const summary = ordersRes.data.map(order => {
const product = inventoryRes.data.find(item => item.id === order.productId);
return {
orderId: order.id,
productName: product ? product.name : 'Unknown',
quantity: order.quantity,
stock: product ? product.stock : 0,
};
});
res.json(summary);
} catch (error) {
console.error('Error fetching data:', error);
res.status(500).json({ error: 'Failed to fetch data' });
}
});
app.listen(PORT, () => {
console.log(`Gateway Service running on <http://localhost>:${PORT}`);
});
Step 2: Building the React Front-End
- Setting Up the React App:
Create a new React app:
npx create-react-app microservices-demo
cd microservices-demo
npm start
Install Axios for API requests:
npm install axios
- App.js:
This React app fetches data from the Gateway Service and displays a summary of orders and inventory.
import React, { useEffect, useState } from 'react';
import axios from 'axios';
const App = () => {
const [summary, setSummary] = useState([]);
const [loading, setLoading] = useState(true);
useEffect(() => {
const fetchData = async () => {
try {
const response = await axios.get('<http://localhost:5003/summary>');
setSummary(response.data);
} catch (error) {
console.error('Error fetching data:', error);
} finally {
setLoading(false);
}
};
fetchData();
}, []);
if (loading) return <p>Loading...</p>;
return (
<div>
<h1>Order Summary</h1>
<table>
<thead>
<tr>
<th>Order ID</th>
<th>Product Name</th>
<th>Quantity</th>
<th>Stock</th>
</tr>
</thead>
<tbody>
{summary.map(item => (
<tr key={item.orderId}>
<td>{item.orderId}</td>
<td>{item.productName}</td>
<td>{item.quantity}</td>
<td>{item.stock}</td>
</tr>
))}
</tbody>
</table>
</div>
);
};
export default App;
Step 3: Running the application
- Start the microservices:
node order-service/index.js
node inventory-service/index.js
node gateway-service/index.js
- Start the React app:
npm start
Result:
When you run the React app, it will display a table summarizing orders and their corresponding product inventory. This demonstrates how React can interact with multiple microservices through a gateway service in a microservices architecture.
Benefits of an API Gateway:
- Single Endpoint: React only needs to communicate with one endpoint, reducing complexity.
- Load Balancing & Caching: Improves performance by distributing traffic and caching responses.
- Security: Centralizes authentication and authorization, ensuring consistent security across services.
5. State Management in React for Microservices Data
As the front-end grows, managing the state of data fetched from multiple microservices becomes crucial. Efficient state management ensures the app remains responsive and consistent, even when dealing with asynchronous data or multiple API responses.
Popular State Management Tools:
- Redux: Centralized store, great for large applications.
- Context API: Built-in React solution for smaller apps.
- React Query: Handles data fetching, caching, and synchronization with minimal boilerplate.
For microservices-heavy applications, React Query is particularly powerful as it abstracts much of the complexity around data fetching and caching.
Using React Query for Microservices Data:
- Install React Query:
npm install @tanstack/react-query
- Setup React Query in App.js:
import React from 'react';
import { useQuery, QueryClient, QueryClientProvider } from '@tanstack/react-query';
import axios from 'axios';
const queryClient = new QueryClient();
// Fetch summary data from the Gateway Service
const fetchSummary = async () => {
const { data } = await axios.get('<http://localhost:5003/summary>');
return data;
};
const App = () => {
const { data: summary, isLoading: summaryLoading } = useQuery(['summary'], fetchSummary);
if (summaryLoading) return <p>Loading...</p>;
return (
<div>
<h1>Order Summary</h1>
<table>
<thead>
<tr>
<th>Order ID</th>
<th>Product Name</th>
<th>Quantity</th>
<th>Stock</th>
</tr>
</thead>
<tbody>
{summary.map(item => (
<tr key={item.orderId}>
<td>{item.orderId}</td>
<td>{item.productName}</td>
<td>{item.quantity}</td>
<td>{item.stock}</td>
</tr>
))}
</tbody>
</table>
</div>
);
};
export default function WrappedApp() {
return (
<QueryClientProvider client={queryClient}>
<App />
</QueryClientProvider>
);
}
Advantages of React Query:
- Automatic Caching: Data is cached and only refetched when necessary.
- Background Refetching: Keeps data up-to-date without manual intervention.
- Optimistic Updates: Updates UI before the server confirms the change, creating a smooth user experience.
6. Challenges and Best Practices
While microservices offer flexibility and scalability, integrating them into a full-stack application with React comes with challenges.
Common Challenges:
- Data Synchronization: Handling asynchronous data from multiple services can lead to race conditions or inconsistent states.
- Service Downtime: If a service is down, the front-end must handle errors gracefully without breaking the user experience.
- Cross-Origin Requests: Dealing with CORS (Cross-Origin Resource Sharing) issues when services are hosted on different domains.
- Monitoring: Monitoring different services could be challenging in some cases
- Inter-service communication: Inter-service communication becomes challenging in large complex applications where different tech stacks are used for different services
Best Practices for React & Microservices Integration:
- Centralized Error Handling: Use React Query’s error boundaries or custom hooks to handle API errors consistently.
- Environment Variables: Use .env files to manage service URLs for different environments (development, staging, production).
- Retry Mechanisms: Implement automatic retries and fallbacks when services fail.
- Lazy Loading: Load data only when needed, especially for non-critical services, to improve performance.
Example of Error Handling with React Query:
const { data, error, isError } = useQuery(['summary'], fetchSummary);
if (isError) {
return <p>Error fetching product summary: {error.message}</p>;
}
7. Conclusion
Microservices have revolutionized full-stack development, offering exceptional flexibility, scalability, and maintainability. React.js, with its modular architecture and robust data handling capabilities, serves as an ideal front-end framework for interfacing with microservices.
In this blog, we've explored how React seamlessly integrates with a microservices architecture—from setting up basic services to handling state and managing API communication through an API Gateway. We've also discussed practical strategies for efficient data management using tools like React Query.
By adopting microservices and leveraging React's strengths, developers can build robust, scalable applications that are easier to maintain and evolve. As you continue exploring this architecture, consider diving deeper into advanced topics like micro-frontends, service orchestration, and containerization with Docker and Kubernetes.