Authentication and authorization using Apollo client and Auth0 in React-GraphQL application
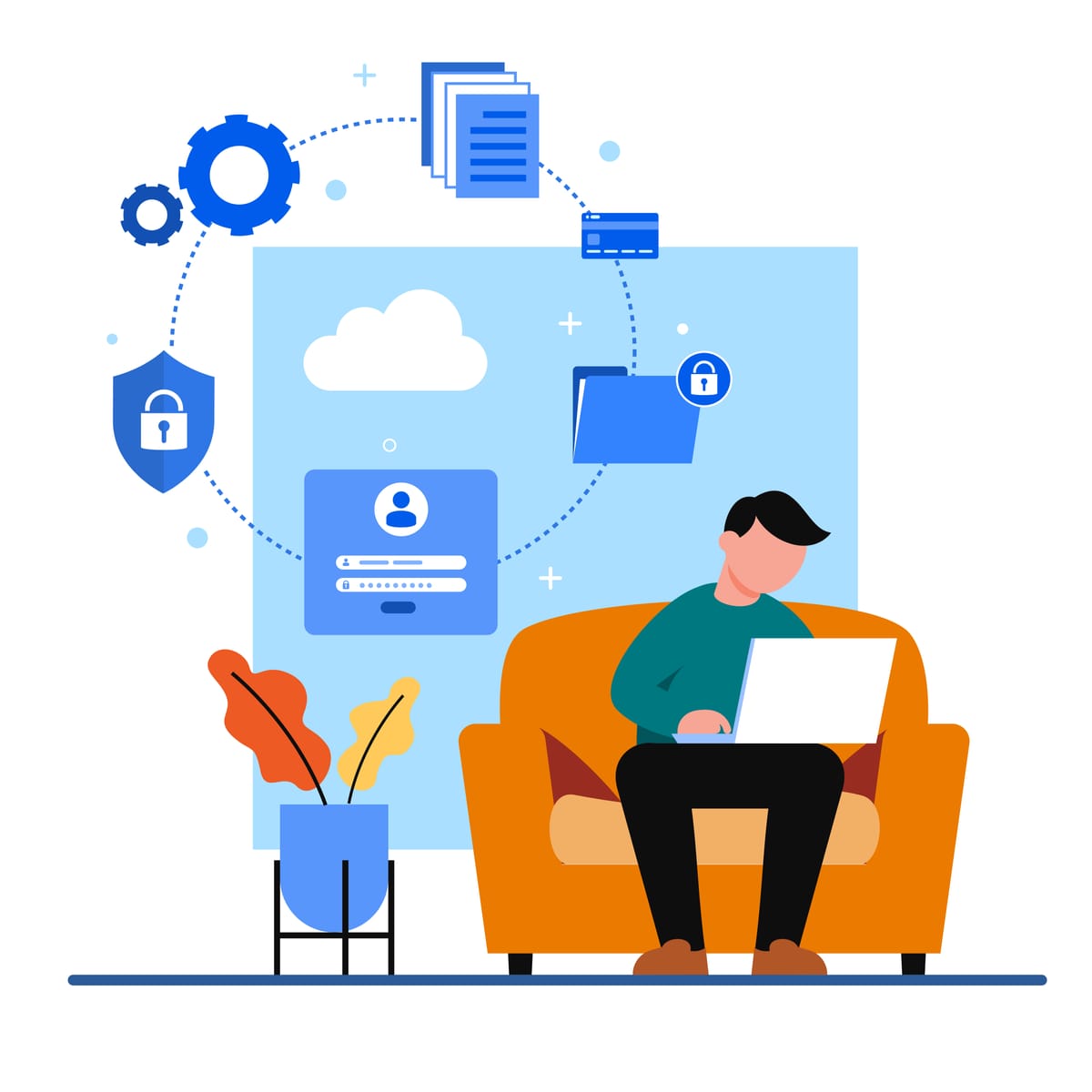
Authentication and authorization are critical for securing web applications, ensuring that only verified users can access resources and perform specific actions. In a React application using Apollo Client for GraphQL interactions, integrating a service like Auth0 simplifies these processes. Auth0, a popular identity management platform, offers features like social login, multi-tenancy, and automatic token refresh, making it ideal for modern applications. In this guide, we’ll explore how to handle authentication and authorization using Auth0, Apollo Client, and GraphQL.
Below, we break down each aspect of setting up authentication and authorization, providing context, potential structure, and supporting details based on recent trends and resources.
Introduction to Authentication and Authorization
- Authentication: Verifies user identity, typically through login credentials or third-party services like Google or Auth0.
- Authorization: Determines user permissions, controlling access to specific data or operations.
- Importance: Ensures security, protects user data, and enhances user experience by restricting unauthorized access.
Why Use GraphQL and Apollo Client?
- GraphQL: Developed by Facebook and open-sourced in 2015, it allows clients to request exact data, reducing over-fetching and under-fetching compared to REST APIs.
- Apollo Client: A comprehensive library for managing GraphQL requests, caching, and state in React applications, enhancing data fetching efficiency.
- Benefits: Simplifies data management, supports real-time updates, and integrates seamlessly with React for dynamic UIs.
Introducing Auth0
- What is Auth0?: A platform for identity management, offering authentication as a service with features like social login, multi-factor authentication, and role-based access control.
- Key Features: Free tier (no credit card required), user database, Google sign-in, custom login pages, and support for refresh token rotation.
- Integration Benefits: Reduces development time, enhances security, and handles token management, including storage in LocalStorage by default.
Setting Up the Project
- Prerequisites:
- Ensure Node.js, npm, and a text editor or IDE are installed. Basic knowledge of React, Apollo Client, and GraphQL is recommended.
- Auth0 Account Setup:
- Sign up at Auth0, select a Personal account.
- Create a Single Page Web Application in the Auth0 dashboard, configuring:
- Allowed Callback URLs (e.g., http://localhost:3000/).
- Allowed Logout URLs, Allowed Origins (CORS), and Grant Types (Implicit, Authorization Code, Refresh Token).
- Create an API with an identifier (e.g., app URL or UUID) and RS256 signing algorithm.
Environment Variables:
- Set in .env
REACT_APP_AUTH0_DOMAIN=yourdomain.auth0.com
REACT_APP_AUTH0_clientID=yourclientid
REACT_APP_API_AUDIENCE=your_api_identifier
Dependencies:
npm install @auth0/auth0-react apollo-client graphql
Handling Authentication with Auth0 in React
Wrap App with Auth0Provider
- Import and use in index.js or main entry file:
import { Auth0Provider } from '@auth0/auth0-react';
const auth0Config = {
domain: process.env.REACT_APP_AUTH0_DOMAIN,
clientId: process.env.REACT_APP_AUTH0_clientID,
redirectUri: window.location.origin,
};
ReactDOM.render(
<Auth0Provider {...auth0Config}>
<BrowserRouter>
<CustomApolloProvider>
<App />
</CustomApolloProvider>
</BrowserRouter>
</Auth0Provider>,
document.getElementById('root')
);
- Ensure Auth0Provider is the outermost wrapper, followed by BrowserRouter, then CustomApolloProvider.
Using useAuth0 Hook
- Provides functions like loginWithRedirect, logout, getAccessTokenSilently, and user data.
- Example for login/logout:
import { useAuth0 } from '@auth0/auth0-react';
function LoginButton() {
const { loginWithRedirect } = useAuth0();
return <button onClick={() => loginWithRedirect()}>Log In</button>;
}
function LogoutButton() {
const { logout } = useAuth0();
return <button onClick={() => logout({ returnTo: window.location.origin })}>Log Out</button>;
}
function Profile() {
const { user } = useAuth0();
if (user) return <div>Welcome, {user.name}</div>;
return <div>Not logged in</div>;
}
- getAccessTokenSilently fetches the access token, handling refresh automatically, which is an unexpected benefit for seamless user experience.
Integrating Authentication with Apollo Client
- Challenge: Apollo Client needs to include the access token in each GraphQL request, but useAuth0 is a hook, limiting its use outside components.
- Solution: Create a CustomApolloProvider component that uses useAuth0 and sets up the client
import React from 'react';
import { ApolloProvider } from '@apollo/react-hooks';
import { useAuth0 } from '@auth0/auth0-react';
import { ApolloClient, InMemoryCache, createHttpLink } from '@apollo/client';
import { setContext } from '@apollo/client/link/context';
function CustomApolloProvider({ children }) {
const { getAccessTokenSilently } = useAuth0();
const getTokenFuncRef = React.useRef(getAccessTokenSilently);
React.useEffect(() => {
getTokenFuncRef.current = getAccessTokenSilently;
}, [getAccessTokenSilently]);
const client = React.useMemo(() => {
const httpLink = createHttpLink({ uri: '/graphql' });
const authLink = setContext(async (request, { headers }) => {
const token = await getTokenFuncRef.current();
return { headers: { ...headers, authorization: `Bearer ${token}` } };
});
const link = authLink.concat(httpLink);
const cache = new InMemoryCache();
return new ApolloClient({ link, cache });
}, []);
return <ApolloProvider client={client}>{children}</ApolloProvider>;
}
export default CustomApolloProvider;
- Explanation: Uses useRef to ensure getAccessTokenSilently is always up-to-date, preventing client recreation on each render by memoizing with an empty dependency array, addressing potential performance issues.
Handling Authorization
- Server-Side Authorization
- On the server, verify the access token using libraries like express-jwt or express-openid-connect with Apollo Server.
- Example setup:
import { ApolloServer } from '@apollo/server';
import express from 'express';
import { expressJwt } from 'express-jwt';
const server = new ApolloServer({
typeDefs,
resolvers,
context: ({ req }) => ({ user: req.user }),
});
const app = express();
app.use(
expressJwt({
secret: jwksClient,
audience: 'your_api_audience',
issuer: `https://${process.env.AUTH0_DOMAIN}/`,
algorithms: ['RS256']
})
);
server.start().then(() => {
server.applyMiddleWare({ app });
app.listen({ port: 4000 }, () => {
console.log('Server listening on port 4000');
});
});
- This ensures requests are authenticated, and user context is available in resolvers for role-based access control.
- Client-Side Conditional Rendering
- Fetch user data via a GraphQL query, e.g.:
query User {
user {
id
name
role
}
}
- Use in a component:
import { useQuery } from '@apollo/react-hooks';
import gql from 'graphql-tag';
const USER_QUERY = gql`
query User {
user {
id
name
role
}
}
`;
function UserProfile() {
const { data, loading, error } = useQuery(USER_QUERY);
if (loading) return <div>Loading...</div>;
if (error) return <div>Error: {error.message}</div>;
return (
<div>
<h2>Welcome, {data.user.name}</h2>
<p>Your role is: {data.user.role}</p>
</div>
);
}
- Conditionally render based on role, e.g., admin panel:
function AdminPanel() {
const { data } = useQuery(USER_QUERY);
if (data && data.user.role === 'admin') return <div>Admin Panel</div>;
return null;
}
- This leverages Apollo Client’s caching for efficiency, ensuring user data is available without refetching.
Best Practices and Security Considerations
- Use HTTPS: Essential for encrypting communication, preventing man-in-the-middle attacks.
- Secure Token Storage: Auth0 stores tokens in LocalStorage by default, which may be vulnerable to XSS; consider secure storage methods if needed.
- Regular Updates: Keep dependencies updated to patch security vulnerabilities, especially for @auth0/auth0-react and apollo-client.
- Avoid Hardcoding: Use environment variables for sensitive information like client IDs and domain names.
- Role-Based Access Control: Implement in resolvers to restrict data access based on user roles, enhancing server-side security.
- Monitor Security: Use tools to monitor for suspicious activities, ensuring timely response to potential breaches.
- Token Refresh: Auth0’s getAccessTokenSilently handles refresh automatically, reducing manual management, which is an unexpected benefit for developers.
Conclusion
By integrating Auth0 with Apollo Client, React, and GraphQL, we ensure secure authentication and fine-grained authorization for our applications. This setup allows for seamless authentication while enforcing role-based access in the backend.
If you’re building production-ready applications, consider adding refresh token management, logging, and additional security measures to strengthen your authentication flow.